RESTFul WebService Development and Consumption With Oracle ADF
Read more...
In the web services world, REpresentational State Transfer (REST) is a key design idiom that embraces a stateless client-server architecture in which the web services are viewed as resources and can be identified by their URLs. The HTTP methods such as GET and POST are the verbs that the developer can use to describe the necessary create, read, update, and delete (CRUD) actions to be performed. Conforming to the REST constraints is referred to as being ‘RESTful’.
This tutorial illustrates how to implement the REST architecture using Oracle JDeveloper 11g 11.1.2. It shows how to create a REST service from simple Java classes and then shows you how to consume and interact with a REST service from an ADF Web application through the use of the URL Service Data Controls.

- To download the Rest.zip file right click the following link and choose Save Link As... from context to download the file on a local directory of your choice.
- Unzip the Rest.zip file on a local directory of your choice (i.e. temp)
- Start JDeveloper by selecting Start > Programs > Oracle Fusion Middleware 11.1.2.0.0 > JDeveloper Studio 11.1.2.0.0If a dialog box opens asking if you would like to import preferences from a previous JDeveloper installation, click NO.
- In the Select Role dialog, choose Studio Developer and click OK.
- Click the Open Application link (or from the tool menu, choose File | Open).
- Locate the workspace Rest.jws where you've unzipped the Rest.zip file. i.e.: c:\temp\Rest.
- Expand RestServiceProject to discover the existing components.
The project contains a Java class called Emp that defines the data structure that we are working with, and another class called Service that provides various methods on the Emp object such as adding and deleting instances of the object. - To add the Jersey library, right-click RestServiceProject and select Project Properties from context .To make your project REST compliant, you need to add the Jersey library.
Read more...
- In the Project Properties dialog, select the Libraries and Classpath node and click the Add/JAR/Directory.
- Expand the Rest | RestServiceProject | public_html | Jersey | directory nodes where you installed the Rest application and select the lib folder.Click Select.
- Back to the Project Properties dialog, the new library has been added, click OK. Then save all your work.JAX-RS: Java API for RESTful Web Services is a Java programming language API that provides support in creating web services according to the Representational State Transfer (REST) architectural style.
Read more...
Shaping, which is based on the role of the user, allows the JDeveloper environment to tailor itself. It does this by removing unneeded items from the menus, preferences, new gallery, and even individual fields on dialogs.
Read more...

Close the Tip of the Day window.
Once loaded, the JDeveloper IDE appears.
Read more...

REST-style architectures consist of clients and servers.
Read more...

To turn your POJO and WebService into REST supporting services you'll use annotations. You are going to map specific methods in your code to specific REST operations by adding the appropriate annotation. We'll also see how annotations are used to map parameters on the request URL to be used in the service.
To make your classes REST compliant, perform the following steps:
- Double click the Emp.java node to open the POJO class in the java code editor and explore its code.
The Emp class is a standard data transfer object with all the getters and setters for an employee object. Later you'll add a tooString method to provide an XML string to represent this object at runtime.The REST architectural style describes the following six constraints applied to the architecture, while leaving the implementation of the individual components free to design.Read more...
- Right above the class definition add the @XmlRootElement annotation statement which defines the root element for a XML tree.
- As requested, press the Alt + Enter keys combination to populate the required import statement.
- At the bottom of the class, add the following method that returns a displayable string for an employee. Then save all your work.public String toString(){
String empXml = ""+getName()+" "+"\n"+
""+getId()+" "+"\n"+
""+getExp()+" "+"\n"+
""+getSalary()+" ";
return empXml;
} - Double click Service.java to open the web service class and explore its code.
The Service class is a class with the CRUD methods using annotation. In the next few steps, you'll add annotations to bind consumer methods to HTTP methods.
Also included in the class is a collection that is defined as a static java collection (static ArrayList).
It adhears to the Singleton design pattern. It indicates there is a single class of this type and there will only ever be one instance of this array in memory. This allows the class to employ stateful behavior. - Prior to the Class definition, enter the following annotation: @Path("/restlab"). The complete path to a web service resource is now based on the base URL and the @Path annotation from your class.
- Click the light bulb in the margin and select the Configure Project for JAX-RS Web Services option.
- While configuring the project with this option, the web.xml and the weblogic.xml files have been created
Close the weblogic.xml tab and looking at the Service.java entry in the Application Navigator, notice that the icon next to the name has changed. The class is now recognized as a web service class type.
Save all your work. - In the Service.java class, above the //Read comment, add the @GET annotation syntax which Indicates that the following method will answer to a HTTP GET request.Press the Alt + Enter key combination to add the proposed import statement.
- Just after the @GET annotation, add @Produces("application/xml"). @Produces defines which MIME type is delivered by a method annotated with @GET.Press the Alt + Enter key combination to add the proposed import statement.
- Change the signature of the addEmp method from:
public void addEmp(int id, int exp, String name, int sal){
to:
public void addEmp(@QueryParam("id") int id, @QueryParam("exp")int exp, @QueryParam("name") String name, @QueryParam("sal")int sal) {The @QueryParam is a parameter that is used in the URL and map to the id variable name.
Press the Alt + Enter key combination to add the proposed import statement. Save all your work. - Just above the //DELETE comment, add @DELETE annotation.Press the Alt + Enter key combination to add the proposed import statement.
- Change the signature for the deleteEmp method adding the @QueryParam annotation:
public void deleteEmp(@QueryParam("id") int id) { - After the Helper Methods comment, add the following annotation and methods to populate employee records.@PUT
@Path("defaultEmp")
public Response addEmp(){
loe.add(new Emp("Adderley",1,5,1000));
loe.add(new Emp("Coltrane",2,6,2000));
loe.add(new Emp("Davis",3,7,3000));
return Response.ok().build();
}Press the Alt + Enter key combination to add the proposed import statements. - Verify that you have the following import statements and then save all your work.import java.util.*;
import javax.ws.rs.DELETE;
import javax.ws.rs.GET;
import javax.ws.rs.PUT;
import javax.ws.rs.Path;
import javax.ws.rs.Produces;
import javax.ws.rs.QueryParam;
import javax.ws.rs.core.Response; - Double click the emp.xsd node to open the xml file and explore it. The XSD file (XML Schema Document) is a schema definition file, one of several XML schema languages. The XSD can be used to express a set of rules to which an XML document must conform in order to be considered 'valid' according to that schema.
Notice the three attributes defined in the emp class (id, name & salary). - Click the Source tab to display the xml code. This representation of the file shows you the detailed definition of the schema, including the elements and element types.
- Double click the emp.xsl node to open the xml file and explore its code. The XSL file (Extensible Stylesheet Language) is used to transform and render XML documents. XSL is a language for expressing style sheets. A XSL style sheet is like a CSS file, a file that describes how to display an XML document of a given type.
- Click the Save All
icon on the JDeveloper menu bar to save your work.
- Right click RestServiceProject and select Rebuild from context.
- Make sure the compilation is successful.
- Right-click the Service.java node in the Application Navigator and select Test Web Service from context.
- Wait for the web service to be deployed. Then the HTTP Analyzer gets uploaded.
- Notice that the Method is already set and the GET Method is specified by default. Click the method list of values to discover all the available methods.
- Switch back to the Service.java class. Notice that the Get method matches the getEmps method in the Service.java class.
- Back in the HTTP Analyzer, click the Send Request button to retrieve the existing employees.No employees are returned yet, since no employees have been populated. Notice the 200 OK return status indicating that the method was successfully performed.
- Click the Service.java tab and locate the PUT annotation where the emps are populated. Notice that the path for the addEmp method is called defaultEmp.
- In the Log window, click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab link to re launch a new request.
- Back to the HTTP Analyzer, add /defaultEmp at the end of the URL and select the PUT method.Click the Send Request button.
- Once again the 200 OK status indicates that the request was performed successfully, meaning that the Emps were created.
- In the Log window re-click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab link to re launch a new request.
- Click the Send Request button to perform the default GET method and fetch the employees previously created.Now, you can see that all employees defined in the PUT annotation are retrieved and displayed.
- In the Log window re click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab link to re launch a new request.
- To test the Delete method, at the end of the URL type: ?id=1 to indicate which specific emp to delete and choose the DELETE method from the list.
Click the Send Request button.The 204 No Content status is now returned. - Back In the Log window re-click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab
link to re launch a new request. Notice the first emp name Adderley is displayed. - Click the Send Request button with the default GET method to fetch the employees. Notice that emp #1 has been removed.
- In the Log window re-click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab link to re launch a new request.
- At the end of the URL type: ?id=2 to indicate which specific emp to delete and choose the DELETE method from the list. Click the Send Request button.
- Back In the Log window re-click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab
link to re launch a new request. - Click the Send Request button with the default GET method to fetch the employees. Notice that emp #2 is gone.
- In the Log window re-click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab link to re launch a new request.
- At the end of the URL type: ?id=3 to indicate which specific emp to delete and choose the DELETE method from the list. Click the Send Request button.
- Back In the Log window re-click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab link to re launch a new request. Click the Send Request button with the default GET method. No more emps are returned.
- Back in the Log window re-click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab link to re launch a new request. Append /defaultEmp at the end of the URL, select the PUT method and click the Send Request button. This is to repopulate the web service with employees again.
- Back In the Log window re-click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab link to re launch a new request. Click the Send Request button with the default GET method. Emps have been repopulated.Now that the emps are populated again, you see how you can use these data in a page.
- Right-click any tab and select Close All from context to dismiss all opened editors.
- Now that the Back-end side is tested and validated, you implement the web service in a Front-end side where web service REST methods can be used as data controls and implemented in a page.
To create the application perform the following steps:
- From the down arrow, select New Application to open the New Gallery.
- In the New Gallery, choose the Fusion Web Application (ADF) and click OK.
Rest
- In the Name your application page of the Create Fusion Web Application wizard, modify the default application name to REST_DC.Click Finish.
- The new ADF Fusion application creates two projects. The Model project and the ViewController project.
- In the Application Navigator, projects are displayed as the second level in the hierarchy under the application. The Application Navigator should look like this:
The application is the highest level in the control structure. It is a view of all the objects you need while you are working. An application keeps track of all your projects while you are developing your Java programs.
Read more...

This step explains how to expose URL services using the ADF Model layer. A URL service can be simply a URL against which a query is posted, so that the URL can be exposed as an ADF form. For example, you can have a URL service that allows you to access employee data for your company.
With RESTFul web services, there is a natural mapping between the HTTP methods and most CRUD-like business operations that many services expose.
Read more...

- Right-click the Model node in the Application Navigator and select New... from the context menu.
- The New Gallery displays. Click the All Features tab, select the Business Tier | Data Controls node as the category and choose URL Service Data Control as the Item. Then click OK.
- In the Create URL Service Data Control dialog, change the name to URL_Get, and have the Connection field set to Create New Connection.Click Next.
- In the Connection step, enter the following values:
Name RESTConn URL Endpoint http://localhost:7101/ Source Rest_lab-RestServiceProject-context-root/jersey/restlab Click Next.In the Parameters step, click Next again. - In the Data Format step, select XML from the drop-down list. In the new fields, enter the following values:
XSD URL: http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/emp.xsd
XSL URL: http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/emp.xslClick Next. - In the Finish step, click the Test URL Connection button. It should return a URL successful status if the web service is up and running.Click Finish.
- The Application Navigator now should look like the following:Notice that the Get method created in the Data Controls accordion.
- The DataControls.dcx opens in the editor showing the URL_Get data control in the Data Control Registry.
Save all your work. - Right-click the Model node again to create a the delete service. In the New Gallery, select the Business Tier | Data Controls node as the category and choose URL Service Data Control as the Item. Then click OK.
- In the Data Source step of the Create URL Service Data Control, type URL_Delete as the name.
Select RESTConn as the Connection from the drop-down list.
Select DELETE as the Http Method.
In the Source, enter Rest_lab-RestServiceProject-context-root/jersey/restlab?id=##id##.Click Next. - In the Parameters step, for the id parameter enter the default value 0.Click Next.
- In the Data Format step, select XML as the Data Format.Click Next.
- In the Finish step click the Test URL Connection button.You should get a URL successful return status. Click Finish.
Save your work. - Open the Data Controls accordion to visualize the created data controls.
Press the refresh icon to see the two new data controls.In the Data Controls panel, each data control object components can create a a different type of component.Read more...
- You can also review the details of the data controls in the editor. Expand the data control nodes to examine each component created.
- Click the Save All
icon on the JDeveloper menu bar to save your work.
The URL service data control enables you to access and consume the data stream from a specified URL.
Read more...

REST services are web services that can be accessed using a simple HTTP URL, rather than a more complex SOAP protocol.
Read more...

The model project is now defined and contains the data control pointing to the web service. Now you can start building a page that implements the data controls. To do so, perform the following actions:
- Collapse the Model project and expand the ViewController one.
- Right-click the ViewController node and select New from context.
- In the New Gallery, select the Web Tier | JSF/Facelets node and select the Page item.You can create UI pages that allow you to display and collect information using data controls created for your business services.
Read more...
Click OK. - In the Create JSF Page dialog, type Emp as the File Name , select Facelets as the Document Type and choosing the Page Template, select the Oracle Three Column Layout.Click OK.
- Wait for the page to display in the Design editor.
- You now have a page with three facets (start - center - end). Scroll to the right and right-click in the end pane and select Delete from context since you will only use two panels.
- In the Data Controls accordion, select the URL_Get | loadData | Return | emps | emp entry and drop it onto the center facet. From the context menu, select Table --> ADF Read-only TableUnlike forms, tables allow you to display more than one data object from a collection at a time.
Read more...
- In the Edit Table Columns, click OK to accept defaults.
- In the Data Controls pane, select the loadData method and drop it above the af:table - t1 in the Structure pane.
- From the context menu select ADF Button.
- In the Structure pane, select the af:table - t1 node and move it up just above the button you just created.
- Select the loadData button and in the Property Inspector, type Query Emps in the text field.
- Collapse the URL_Get node and expand the URL_Delete one.
- Select the loadData (String) entry and drop it onto the start facet.
- Select ADF Parameter Form from context.Instead of dropping each of the individual attributes of a collection to create a form, you can a complete form that displays or collects data for all the attributes on an object.
Read more...
- In the Edit Form Fields type Emp Id in the Display Label for the id component.Click OK.
- Select the loadData2 button and in the Property Inspector, enter Delete Emp as the Text.
- Your page should look like the following:
- Click the Save All
icon on the JDeveloper menu bar to save your work.
In this step, you run the page and experiment the REST methods implemented as ADF data controls. In order to test the page, the WebLogic Server must be started and the employee collection must be loaded. If your environment is not setup in this way, complete the first three steps. Otherwise you may skip them and start on number 4.
- Right-click the Service.java node in the Application Navigator and select Test Web Service from context.
- In the Log window re-click the http://127.0.0.1:7101/Rest_lab-RestServiceProject-context-root/jersey/restlab link to re launch a new request.
- In the HTTP Analyser, a ppend /defaultEmp at the end of the URL, select the PUT method and click the Send Request button. This is to repopulate the web service with employees again.
- In the Application Navigator, right-click the Emp.jsf node and select Run from context.
- Wait for the page to display in your browser.Notice that the Emps are queried automatically displaying the existing records.
- In the Delete Emp section, enter a value for an existing emp id to delete. (i.e. 2).
- From the center pane, click the Query Emps button.Notice that one of the emp has been removed from the list.
- In the right side pane, enter a new id value (3) and click the Delete Emp button.
- Back in the center pane, click the Query Emps button. The deleted employee is now gone.
- You can try to remove the last employee, if you wish.
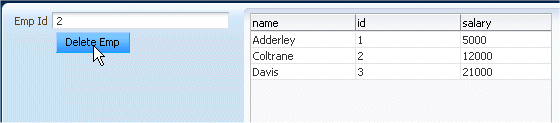
Click the Delete Emp button.
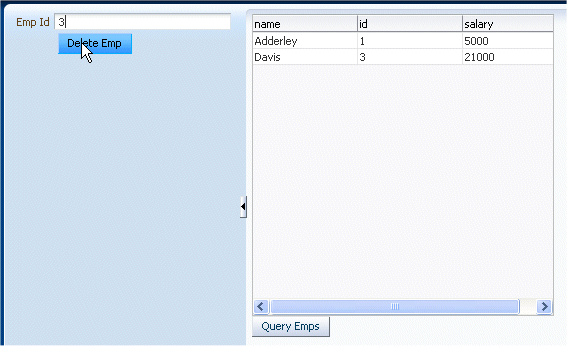
You've successfully performed this REST tutorial.
Summary
In this tutorial you learned how to find your way around the JDeveloper IDE. You learned how to:
- Create Resource and Service classes using REST annotations
- Use the HTTP Analyzer to test a web service
- Create Data Controls based on a REST web service
- Create a JSF page that uses web service data controls
- Run a page to test the REST web service
- Oracle® Fusion Middleware User's Guide for Oracle JDeveloper
11g Release 2 (11.1.2.0.0) - Using the HTTP Analyzer with RESTful Web Services - Oracle® Fusion Middleware User's Guide for Oracle JDeveloper
11g Release 2 (11.1.2.0.0) - Creating RESTful Web Services - RESTful Web Services
1 comment:
I really appreciate information shared above. It’s of great help. If someone want to learn Online (Virtual) instructor lead live training in Oracle JDeveloper 11g Fusion, kindly contact us http://www.maxmunus.com/contact
MaxMunus Offer World Class Virtual Instructor led training on Oracle JDeveloper 11g Fusion. We have industry expert trainer. We provide Training Material and Software Support. MaxMunus has successfully conducted 100000+ trainings in India, USA, UK, Australlia, Switzerland, Qatar, Saudi Arabia, Bangladesh, Bahrain and UAE etc.
For Demo Contact us.
Nitesh Kumar
MaxMunus
E-mail: nitesh@maxmunus.com
Skype id: nitesh_maxmunus
Ph:(+91) 8553912023
http://www.maxmunus.com/
Post a Comment