How do I read from an Excel file using POI?
Author: Deron Eriksson
The Apache Jakarta POI project can be found at http://jakarta.apache.org/poi/. Within the POI project, POI-HSSF focuses on Excel documents. The HSSF Quick Guide at http://jakarta.apache.org/poi/hssf/quick-guide.html is a great resouce for quickly getting up to speed with POI-HSSF.
In this tutorial I'll use the project structure that we created for the other tutorial. This project contains the POI jarW file in its classpathW. We will create a class that reads in data from an Excel file and displays it. The Excel file that we will use is the 'poi-test.xls' file that we previously created.
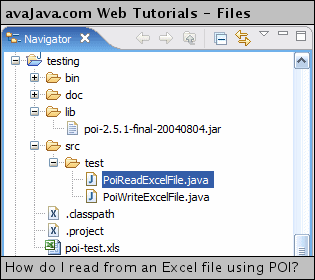
The poi-test.xls file is located at the root level of our project and is shown here:
poi-test.xls
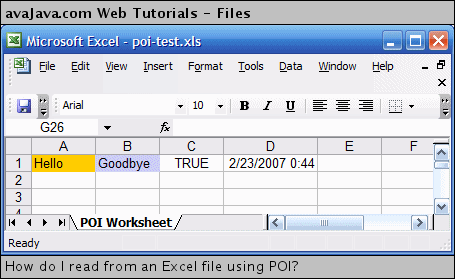
The PoiReadExcelFile class will read in the 'poi-test.xls' file into an HSSFWorkbook object. The 'POI Worksheet' will then be read into an HSSFWorksheet object, and then the values within the A1, B1, C1, and D1 cells will be read and displayed to standard output.
PoiReadExcelFile.java
package test; import java.io.FileInputStream; import java.io.FileNotFoundException; import java.io.IOException; import java.util.Date; import org.apache.poi.hssf.usermodel.HSSFCell; import org.apache.poi.hssf.usermodel.HSSFRow; import org.apache.poi.hssf.usermodel.HSSFSheet; import org.apache.poi.hssf.usermodel.HSSFWorkbook; public class PoiReadExcelFile { public static void main(String[] args) { try { FileInputStream fileInputStream = new FileInputStream("poi-test.xls"); HSSFWorkbook workbook = new HSSFWorkbook(fileInputStream); HSSFSheet worksheet = workbook.getSheet("POI Worksheet"); HSSFRow row1 = worksheet.getRow(0); HSSFCell cellA1 = row1.getCell((short) 0); String a1Val = cellA1.getStringCellValue(); HSSFCell cellB1 = row1.getCell((short) 1); String b1Val = cellB1.getStringCellValue(); HSSFCell cellC1 = row1.getCell((short) 2); boolean c1Val = cellC1.getBooleanCellValue(); HSSFCell cellD1 = row1.getCell((short) 3); Date d1Val = cellD1.getDateCellValue(); System.out.println("A1: " + a1Val); System.out.println("B1: " + b1Val); System.out.println("C1: " + c1Val); System.out.println("D1: " + d1Val); } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
if we execute our PoiReadExcelFile class, we see the following.

7 comments:
Inspiring writings and I greatly admired what you have to say , I hope you continue to provide new ideas for us all and greetings success always for you..Keep update more information..
Data Science Training in Chennai | Best Data science Training in Chennai | Data Science training in anna nagar | Data science training in Chennai
Data Science training in chennai | Best Data Science training in chennai | Data science training in Bangalore | Data Science training institute in Bangalore
Data Science training in marathahalli | Data Science training in Bangalore | Data Science training in btm layout | Data Science Training in Bangalore
Inspiring writings and I greatly admired what you have to say , I hope you continue to provide new ideas for us all and greetings success always for you..Keep update more information..
Java training in Chennai | Java training in Tambaram | Java training in Chennai | Java training in Velachery
Java training in Chennai | Java training in Omr | Oracle training in Chennai
Excellent post!!!. The strategy you have posted on this technology helped me to get into the next level and had lot of information in it.
Data Science Training in Indira nagar | Data Science Training in btm layout
Python Training in Kalyan nagar | Data Science training in Indira nagar
Data Science Training in Marathahalli | Data Science training in Bangalore | Data Science Training in BTM Layout | Data Science training in Bangalore
Great Article… I love to read your articles because your writing style is too good, its is very very helpful for all of us and I never get bored while reading your article because, they are becomes a more and more interesting from the starting lines until the end.
advanced excel training in bangalore | Devops Training in Chennai
I simply wanted to write down a quick word to say thanks to you for those wonderful tips and hints you are showing on this site.
aws Training in indira nagar | Aws course in indira Nagar
selenium Training in indira nagar | Best selenium course in indira Nagar | selenium course in indira Nagar
python Training in indira nagar | Best python training in indira Nagar
datascience Training in indira nagar | Data science course in indira Nagar
devops Training in indira nagar | Best devops course in indira Nagar
It's a wonderful post and very helpful, thanks for all this information about Java. You are including better information regarding this topic in Java training in Chennai
Java Online training in Chennai
Java Course in Chennai
Best JAVA Training Institutes in Chennai
Java training in Bangalore
Java training in Hyderabad
Java Training in Coimbatore
Java Training
Java Online Training
an effective way.Thank you so much.
thank you for sharing this article with us!Your tipps are very helpful. :)
Thank you
Post a Comment